PartOperation
An abstract class that all parts based on solid modeling inherit from.
Summary
Properties
- read parallel
The level of detail used to render the solid modeled part.
- read parallel
An angle in degrees which affects the smooth shading of a solid modeled part.
- read onlynot replicatedread parallel
The number of polygons in this solid model.
- read parallel
Sets whether the PartOperation can be recolored using inherited color properties.
- not replicatedread parallel
Determines the level of detail the part's physics will adhere to its mesh.
- not replicatedread parallel
Determines the geometric representation used to compute aerodynamic forces and torques.
Determines whether a part is immovable by physics.
- not replicatedread parallel
The angular velocity of the part's assembly.
- read onlynot replicatedread parallel
The center of mass of the part's assembly in world space.
- not replicatedread parallel
The linear velocity of the part's assembly.
- read onlynot replicatedread parallel
The total mass of the part's assembly.
- read onlynot replicatedread parallel
A reference to the root part of the assembly.
- read parallel
Determines the type of surface for the Back face of a part (+Z direction).
- read parallel
Determines the type of surface for the Bottom face of a part (-Y direction).
- not replicatedread parallel
Determines the color of a part.
Determines the position and orientation of the BasePart in the world.
- read parallel
Determines whether a part may collide with other parts.
Determines whether the part is considered during spatial query operations.
Determines if Touched and TouchEnded events fire on the part.
- read parallel
Determines whether or not a part casts a shadow.
- read onlynot replicatedread parallel
Describes the world position in which a part's center of mass is located.
- not replicatedread parallel
Describes the name of a part's collision group.
Determines the color of a part.
- read onlynot replicatedread parallel
Indicates the current physical properties of the part.
- read parallel
Determines several physical properties of a part.
- read parallel
Used to enable or disable aerodynamic forces on parts and assemblies.
- read onlynot replicatedread parallel
- read onlynot replicatedread parallel
The actual physical size of the BasePart as regarded by the physics engine.
- read parallel
Determines the type of surface for the Front face of a part (-Z direction).
- read parallel
Determines the type of surface for the Left face of a part (-X direction).
- hiddennot replicatedread parallel
Determines a multiplier for BasePart.Transparency that is only visible to the local client.
Determines whether a part is selectable in Studio.
Describes the mass of the part, the product of its density and volume.
Determines whether the part contributes to the total mass or inertia of its rigid body.
- read parallel
Determines the texture and default physical properties of a part.
- not replicatedread parallel
The name of MaterialVariant.
- hiddennot replicatedread parallel
Describes the rotation of the part in the world.
- read parallel
Specifies the offset of the part's pivot from its CFrame.
Describes the position of the part in the world.
- hiddenread onlynot replicatedread parallel
Time since last recorded physics update.
- read parallel
Determines how much a part reflects the skybox.
- read onlynot replicatedread parallel
Describes the smallest change in size allowable by the Resize method.
- read onlynot replicatedread parallel
Describes the faces on which a part may be resized.
- read parallel
Determines the type of surface for the Right face of a part (+X direction).
- read parallel
The main rule in determining the root part of an assembly.
The rotation of the part in degrees for the three axes.
Determines the dimensions of a part (length, width, height).
- read parallel
Determines the type of surface for the Top face of a part (+Y direction).
- read parallel
Determines how much a part can be seen through (the inverse of part opacity).
- not replicatednot scriptableread parallel
Methods
Substitutes the geometry of this PartOperation with the geometry of another PartOperation.
Apply an angular impulse to the assembly.
Apply an impulse to the assembly at the assembly's center of mass.
Apply an impulse to the assembly at specified position.
- write parallel
Returns whether the parts can collide with each other.
Checks whether you can set a part's network ownership.
- write parallel
Returns a table of parts connected to the object by any kind of rigid joint.
Return all Joints or Constraints that is connected to this Part.
Returns the value of the Mass property.
- write parallel
Returns the current player who is the network owner of this part, or nil in case of the server.
- write parallel
Returns true if the game engine automatically decides the network owner for this part.
- write parallel
Returns the base part of an assembly of parts.
Returns a table of all BasePart.CanCollide true parts that intersect with this part.
- write parallel
Returns the linear velocity of the part's assembly at the given position relative to this part.
- write parallel
Returns true if the object is connected to a part that will hold it in place (eg an BasePart.Anchored part), otherwise returns false.
Changes the size of an object just like using the Studio resize tool.
Sets the given player as network owner for this and all connected parts.
Lets the game engine dynamically decide who will handle the part's physics (one of the clients or the server).
- IntersectAsync(parts : Objects,collisionfidelity : Enum.CollisionFidelity,renderFidelity : Enum.RenderFidelity):Instanceyields
Creates a new IntersectOperation from the overlapping geometry of the part and the other parts in the given array.
- SubtractAsync(parts : Objects,collisionfidelity : Enum.CollisionFidelity,renderFidelity : Enum.RenderFidelity):Instanceyields
Creates a new UnionOperation from the part, minus the geometry occupied by the parts in the given array.
- UnionAsync(parts : Objects,collisionfidelity : Enum.CollisionFidelity,renderFidelity : Enum.RenderFidelity):Instanceyields
Creates a new UnionOperation from the part, plus the geometry occupied by the parts in the given array.
Gets the pivot of a PVInstance.
Transforms the PVInstance along with all of its descendant PVInstances such that the pivot is now located at the specified CFrame.
Events
Events inherited from BasePartFires when a part stops touching another part as a result of physical movement.
Fires when a part touches another part as a result of physical movement.
Properties
RenderFidelity
This property determines the level of detail that the solid modeled part will be shown in. It can be set to the possible values of the Enum.RenderFidelity enum.
By default, solid modeled parts will always be shown in precise fidelity, no matter how far they are from the camera. This improves their appearance when viewed from any distance, but if a place has a large number of detailed solid modeled parts, it may reduce overall performance.
Distance From Camera | Render Fidelity |
---|---|
Less than 250 studs | Highest |
250-500 studs | Medium |
500 or more studs | Lowest |
SmoothingAngle
This property represents an angle in degrees for a threshold value between face normals on a solid modeled part. If the normal difference is less than the value, normals will be adjusted to smooth the difference. While a value between 30 and 70 degrees usually produces a good result, values between 90 and 180 are not recommended as they may cause a "shadowing" effect on unions with sharp edges.
Note that smoothing does not affect the normals between different materials or different colors.
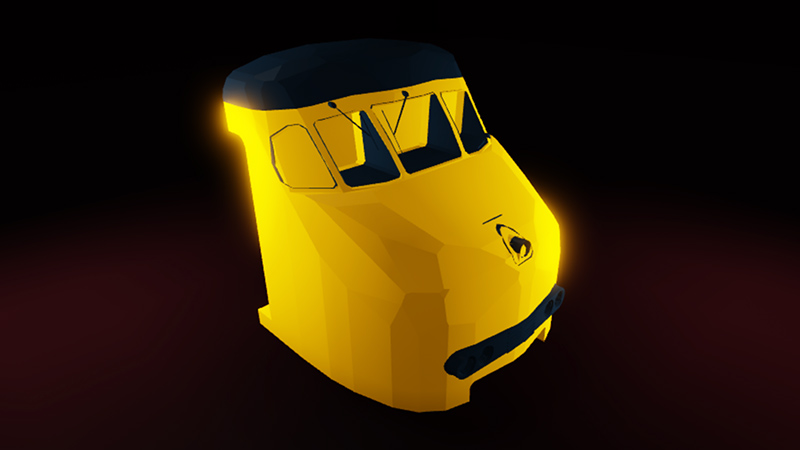
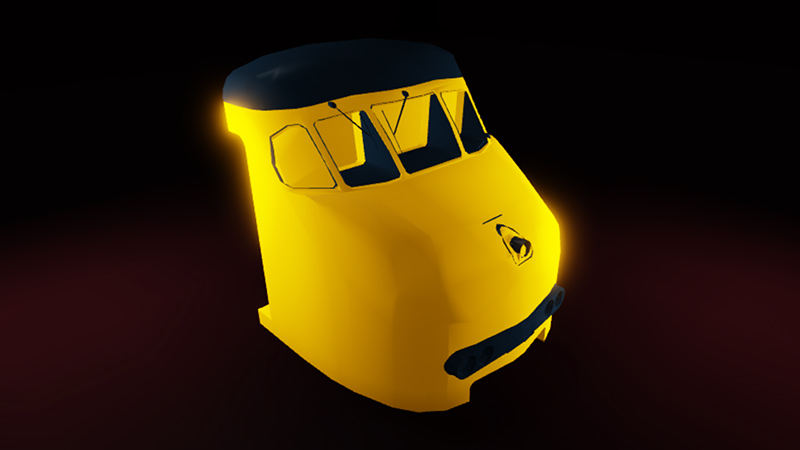
UsePartColor
Sets whether the PartOperation can be recolored using the BasePart.Color or BasePart.BrickColor properties. When true, the entire union will be colored as per Color or BrickColor. When false, the parts in the union will maintain their original colors from before the onion operation was performed.
Methods
SubstituteGeometry
Substitutes the geometry of this PartOperation with the geometry of another PartOperation. This makes it easier to utilize the geometry of a solid modeling operation like UnionAsync(), SubtractAsync(), or IntersectAsync() but maintain properties, attributes, tags, and children of the main part such as Attachments, Constraints, ParticleEmitters, light objects, decals, and more. This approach also circumvents the potential "flicker" of completely replacing the original PartOperation with another.
Note that if you're calling this method on a PartOperation with child Attachments or Constraints, you should calculate the affected instances with CalculateConstraintsToPreserve(), then drop those whose recommended parent is nil.
Parameters
The PartOperation whose geometry will substitute the geometry of this PartOperation.
Returns
Code Samples
local GeometryService = game:GetService("GeometryService")
local mainPart = workspace.PurpleBlock
local otherParts = {workspace.BlueBlock}
local options = {
CollisionFidelity = Enum.CollisionFidelity.Default,
RenderFidelity = Enum.RenderFidelity.Automatic,
SplitApart = false
}
local constraintOptions = {
tolerance = 0.1,
weldConstraintPreserve = Enum.WeldConstraintPreserve.All
}
-- Perform union operation in pcall() since it's asyncronous
local success, newParts = pcall(function()
return GeometryService:UnionAsync(mainPart, otherParts, options)
end)
if success and #newParts > 0 and mainPart:IsA("PartOperation") then
-- Set first part in resulting operation as part to use for substitution
-- First part is simply an option; this can be any PartOperation
local substitutePart = newParts[1]
-- Reposition part to the position of main part
substitutePart.CFrame = mainPart.CFrame
-- Calculate constraints/attachments to either preserve or drop
local recommendedTable = GeometryService:CalculateConstraintsToPreserve(mainPart, newParts, constraintOptions)
-- Substitute main part's geometry with substitution geometry
mainPart:SubstituteGeometry(substitutePart)
-- Drop constraints/attachments that are not automatically preserved with substitution
for _, item in pairs(recommendedTable) do
if item.Attachment then
if item.ConstraintParent == nil then
item.Constraint.Parent = nil
end
if item.AttachmentParent == nil then
item.Attachment.Parent = nil
end
elseif item.WeldConstraint then
if item.Parent == nil then
item.WeldConstraint.Parent = nil
end
end
end
-- Destroy other parts
for _, otherPart in pairs(otherParts) do
otherPart.Parent = nil
otherPart:Destroy()
end
end